How To Use An API In Python
APIs act like bridges. They connect different software systems. Imagine a bustling city with roads. Each road enables travel between places. APIs let programs communicate without hassle. They share data and features smoothly. A world without APIs would be chaotic.
Think about how apps on your phone work together. One app checks the weather. Another delivers your favorite food. Each one relies on APIs.
APIs use specific rules to share information. This structure is like a recipe. It ensures everyone operates the same way. Various types of APIs exist. They include web APIs and library APIs, and more.
Web APIs are crucial for internet apps. They use HTTP requests and responses. Library APIs provide code libraries to developers. They simplify tasks in programming languages.
- APIs save time. They let developers avoid creating everything from scratch.
- APIs allow different systems to work together. This collaboration is key.
- APIs are critical for modern apps. They enhance functionality and user experience.
Most importantly, APIs are user-friendly. They help programmers focus on important tasks. APIs in Python are especially interesting. Python’s simplicity makes API integration easier. Many libraries are available. Requests is a popular choice for making HTTP requests.
Using APIs with Python opens up possibilities. You can access vast data and services. Imagine using Twitter’s API to analyze trends. This is how APIs power our daily tech wonders.
APIs stretch across sectors. Health, finance, and entertainment all use them. They help businesses grow and innovate. Without APIs, progress would slow.
To dive deeper into the fascinating world of APIs and learn best practices, check out our guide on utilizing API integration.
In conclusion, APIs are invisible bridges. They support modern programming. They provide connections that drive innovation. In Python, they create endless opportunities.
Table of Contents
- Setting Up Your Python Environment
- Making Your First API Call
- Authentication Methods: Unlocking the Gates
- Parsing API Responses: Making Sense of the Chaos
- Common Pitfalls to Avoid When Using APIs
- Conclusion: The Future of API Integration in Python
Setting Up Your Python Environment

To begin, you need Python installed on your computer. Head to the official Python website. Download the latest version of Python. Follow the installation prompts. Make sure to check “Add Python to PATH.”
After installation, open your command line or terminal. You’ll want to install libraries to make API calls. Libraries simplify tasks and save time. The two main libraries here are requests and json.
Installing Required Libraries
Installing libraries is simple. Use the command line for this. Type pip install requests and hit enter. You should see a message that confirms installation. Next, type pip install json. This will ensure you have the right tools.
For API calls, the requests library is key. It makes handling HTTP requests easier. JSON is used for data interchange. Both libraries are crucial for beginners.
Configuring Your Environment
- Make a new folder for your project.
- Create a new Python file for your script.
- Open the file in your preferred code editor.
Now includes the libraries in your code. At the top of your Python file, write import requests. Then, add import json. With this, your environment is ready.
In closing, check everything works. Run a test script to see if the libraries load. Mistakes happen. It’s okay to be confused at times. Just keep trying. Follow these steps. You will be one step closer to using APIs in Python. Remember to explore more as you learn.
Making Your First API Call
API calls can seem pretty daunting. Nevertheless, they are not as hard as they look. Let’s take a simple approach. We’ll use the “Dog CEO” API that serves up random dog images. What could be more fun than that?
First, you need to get your tools ready. Make sure you have Python installed. Next, open your favorite code editor. You’ll want to write some Python code. Now, let’s start coding! First, import the `requests` library. This library helps us make API calls easily. You can do this by typing:
import requests
Next, we construct the API request! This is where the fun begins. Use the following URL:
url = "https://dog.ceo/api/breeds/image/random"
This URL points to a public API endpoint! It will give you a random dog image link. Now, it’s time to make the request. Use the `get` function. It retrieves data from the API. Write this line of code:
response = requests.get(url)
Now, here’s the exciting part! You’ve made your first API call. Moving on, we need to check the response. Check if the call was successful. You can do this by looking at the status code. Use:
print(response.status_code)
A code of **200** means everything went well. If it’s not 200, something went wrong! Next, we want to see the data. Let’s convert the response to JSON format. This makes it easy to work with.
data = response.json()
Now, you can extract the image link! Simply navigate through the JSON structure. For that, use:
image_url = data["message"]
What you have now is a text link to a dog image. Use this link to show the image! Open it in your web browser. You should see an adorable dog! Congratulations! You’ve just made your first API call.
Each step is crucial. Remember to pay attention. It may seem simple, but understanding each part is key to improving.
Authentication Methods: Unlocking the Gates
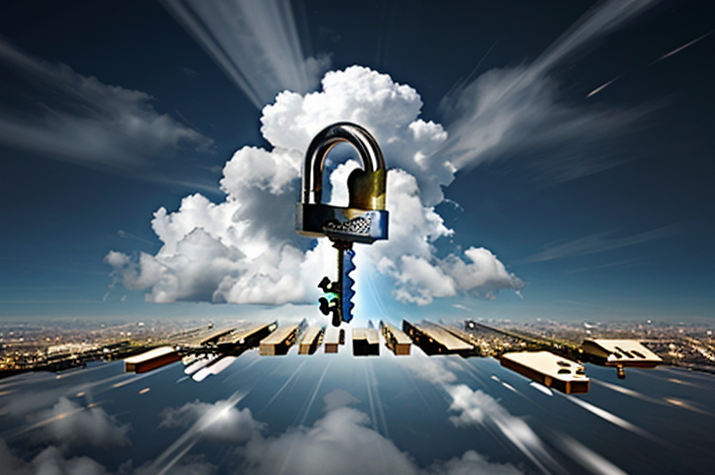
APIs are like doors to valuable data. Without proper keys, those doors stay locked tight. Different authentication methods ensure only the right people gain access. One common method is the API key.
Think of an API key as a secret password. Every time you want to enter a restricted site, the key is required. Without it, the user stands outside looking in, locked out from the treasures that lie beyond.
- OAuth is another method.
- This is like getting a special invitation to an exclusive party.
The host checks your ID before you enter. OAuth allows apps to access your data without sharing your password. Users can feel safe knowing their information stays secure.
Imagine a school. It has many classrooms, each with its own rules. An API acts like the school office. The office gives out access passes. These passes let students in different classrooms. The passes represent tokens.
These tokens are temporary and change often. Just like a student needs a new pass each day. This keeps intruders from staying too long, protecting hard-earned knowledge.
- Make sure you never share your API key.
- Use HTTPS to secure your communication with the API.
- Regularly change your tokens to prevent unauthorized access.
Sometimes developers make mistakes. They forget to read the API documentation. This can lead to using outdated keys. Outdated keys can result in denied access.
Recognizing these methods helps developers. It protects data and keeps systems running smoothly. Problems rarely occur because of proper authentication.
Imagine a magical vault that keeps your information safe. Using strong authentication is like using a powerful spell. It’s essential to keep everything secure. As technology grows, so must our methods of safety.
APIs play vital roles in digital life. Authentication keeps everyone honest. In a world full of data, knowing the right method makes all the difference.
Parsing API Responses: Making Sense of the Chaos
When using APIs, you get data back. Data usually comes in specific formats. The two main formats are JSON and XML. These formats help us understand data better. Imagine them as different languages for information.
JSON means JavaScript Object Notation. It is easy to read and write. Data is organized as key-value pairs. For example: {“name”: “John”, “age”: 30}. This simple structure makes it popular. JSON works well with Python too.
XML, or Extensible Markup Language, uses tags. Tags define pieces of data. It looks more complex than JSON. For instance: John30. XML is great for complicated data. It provides more detail but can be tricky to read.
Both formats have strengths and weaknesses. JSON is easier for most developers. XML does allow for more detailed data description. Sometimes, APIs offer data in both formats. You can choose which to prefer based on the project.
Parsing JSON in Python is simple. You start with importing a library called json. Use the command: import json. Next, you can convert JSON strings to Python dictionaries. Just use json.loads().
For example: data = json.loads(response_text). This will convert your JSON string into a dictionary. You now access data easily. For instance, print(data[‘name’]) displays ‘John’.
Parsing XML takes a bit more effort. You often use the xml.etree.ElementTree module. First, you must import it. Then, you load the XML string with ElementTree.fromstring(). This function creates a tree structure with your data.
Accessing data from XML is different. You navigate through the tree. For example, root.find(‘name’).text gets you the name. This method helps you handle more complex data. It can be confusing, yet rewarding.
Challenges arise when dealing with bad data. Sometimes APIs return errors or empty fields. Always check for errors first. Develop good habits by validating your data. Here are some tips for success:
- Familiarize yourself with JSON and XML.
- Always check for error messages in responses.
- Practice parsing different types of example data.
API responses are like treasures. You unearth valuable information from chaos. Remember this: data is powerful. With practice, parsing becomes second nature.
Common Pitfalls to Avoid When Using APIs
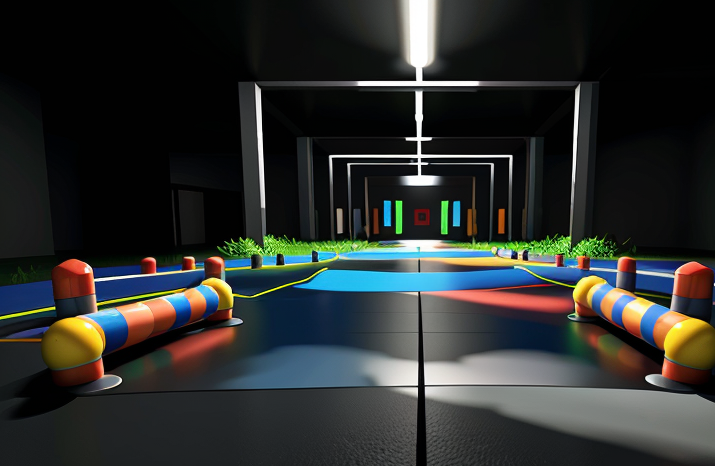
APIs have their quirks. Developers often hit roadblocks along the way. One common pitfall is rate limiting. Imagine building an app that connects to a weather service. Suddenly, you get an error. You realize you’ve made too many requests!
APIs limit the number of calls to keep their service running smoothly. This mistake can slow down your progress. Be careful to track your API usage!
- Watch API documentation closely. It warns you about limits.
- Use backoff strategies if you get rate limited.
Another mistake is misunderstanding responses from the API. Sometimes they return errors in unexpected formats. This happened to a friend of mine. He didn’t check the response code. He assumed success. But the code meant “not found.” This confusion wastes time and effort.
Always inspect the response for status codes.
- Check for 200 OK. It means your call worked.
- Beware of 404 or 500. They signal problems.
Additionally, many miss headers in API responses. Headers provide vital information. For example, your response may include limits for the current request. Ignoring them can leads to mistakes. Make headers a part of your response checks. Sometimes, developers overlook authentication.
APIs require you to prove your identity. Forget your API key, and your requests will be denied. Always store keys securely and access them with care.
- Use environment variables.
- This keeps your keys and tokens hidden.
Another common issue is poor error handling. Diving into a sea of complex code can be challenging. Don’t assume everything will work on the first try. Catch errors in your code. Print out logs to understand what happens. Lastly, testing APIs can feel tricky.
Don’t neglect testing API calls. Sometimes, it’s like going blindfolded. Use tools like Postman for trial runs. These common issues can derail your project. Take them seriously to succeed with APIs!
Conclusion: The Future of API Integration in Python
APIs are changing the world of programming. They create new possibilities for developers everywhere. As technology grows, APIs will become even more important. Imagine connecting different systems seamlessly. This allows for quick data sharing.
- More companies will rely on APIs.
- They enable faster innovation.
- APIs will drive better user experiences.
Many trends emerge in this API landscape. For instance, voice assistants increasingly use APIs. Also, Internet of Things (IoT) tech constantly connects via APIs. They serve as the backbone for various smart devices. In education, APIs will enrich learning tools.
Students will access diverse resources easily, leaning into collaborative learning. New opportunities can arise. With simpler integrations, creativity expands. Imagine what a child could create with powerful API tools. APIs bridge knowledge and applications that excite us.
Many challenges exist, however, like security concerns in connections. It is crucial for developers to ensure data safety. The future holds promise. API integration will continue to grow in creativity and utility. As we look forward, who knows what advancements await us?