Comprehensive OpenGL API Tutorial: A Beginner’s Guide
Before OpenGL, each computer needed its method to draw images. This created confusion and wasted time. Industry pioneers like Mark Segal saw this need for standardization. They worked on what would become OpenGL. This API created a universal way to talk to graphics hardware. It became the lifeline for many graphical applications.
In the beginning, graphics programming was challenging. Developers faced many problems creating visuals. They had to write complex code, which was often slow. Then, in the late 1980s, OpenGL arrived. This new tool changed everything. It made rendering 2D and 3D graphics easier.
OpenGL quickly gained popularity. Games were among the first to use it. Titles like Quake and Doom showcased powerful 3D graphics. Developers loved its ease of use. The benefits stretched beyond just gaming. Scientists used OpenGL for simulations and models.
- OpenGL had many advantages:
- Standardization across platforms.
- Support for real-time rendering.
- Compatibility with many different devices.
- Rich community and resources.
As with anything new, there were bumps along the way. Some developers hesitated to switch to OpenGL. They worried about learning a new system. Yet, they soon realized the rewards. OpenGL offered a pathway to stunning visuals.
Over the years, OpenGL evolved. It added features and improved efficiency. It supported shaders, textures, and lighting. This evolution helped it stay relevant in a fast-paced industry.
OpenGL’s significance is huge. Both gamers and scientists have relied on it for decades. Its impact shapes our visual experiences. OpenGL set the stage for modern graphics programming. Without it, the digital landscapes would be less vibrant.
To further enhance your understanding of software development principles, check out our article on web services.
As we dive deeper into this tutorial, understand this foundation. OpenGL is the key to unlocking graphics potential.
Table of Contents
- Setting Up Your Development Environment
- The Core Concepts of OpenGL: A Primer
- Creating Your First OpenGL Application: A Step-by-Step Tutorial
- Common Challenges and Solutions in OpenGL Development
- The Future of OpenGL and Graphics Programming
Setting Up Your Development Environment
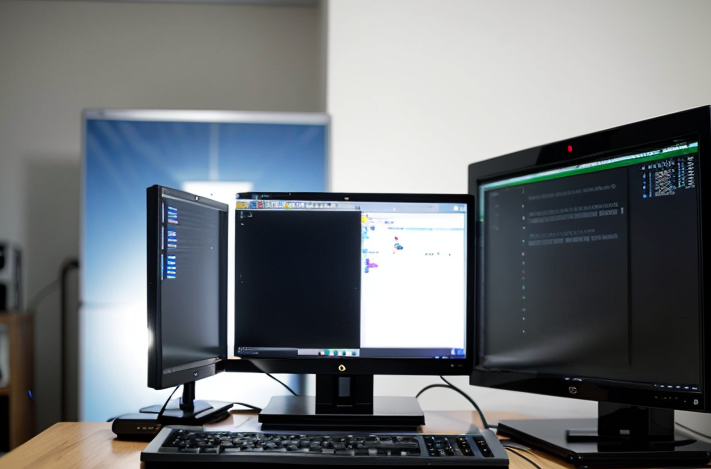
Installing OpenGL is simple. You need an IDE first. IDE stands for Integrated Development Environment. It helps you write and manage your code.
- Popular choices include Visual Studio, Code::Blocks, and Eclipse.
- Choose one that fits your needs. You can download these from their official websites.
Next, you need to install the necessary libraries. Libraries contain code that helps your program work. OpenGL needs libraries to run properly.
For Windows users, GLEW (OpenGL Extension Wrangler Library) is essential. It helps access the newest OpenGL features. You can find GLEW on its official site. For Linux, you often use package managers. Type “sudo apt-get install libglew-dev” in your terminal. This installs GLEW quickly.
After GLEW, you should get GLFW. It manages windows and inputs. Look up “GLFW download” to get it.
Installing Drivers
Drivers are crucial for graphics rendering. They translate code into display. Make sure your graphics card drivers are up to date. Go to the manufacturer’s site like Nvidia or AMD. They have the latest drivers for download. This step is vital for performance.
- For Nvidia: Visit Nvidia’s website.
- For AMD: Head to AMD’s website.
- Install the driver according to the instructions provided.
If you face issues, check these troubleshooting tips. Sometimes, your device needs a restart after installation. Ensure you have set proper environment variables. This helps the system find your installed libraries. Don’t worry if it feels confusing. Each step you take makes it clearer.
Finally, create a simple project in your IDE. This tests if everything is working. Write a small “Hello, World!” code snippet. Run your project. If it runs successfully, you set it up correctly!
Finding community forums helps if stuck. Experienced developers offer great advice. Stay patient and keep trying! Your journey into OpenGL is just beginning. With persistence, you can master it.
The Core Concepts of OpenGL: A Primer
OpenGL is a powerful tool for graphics programming. It represents how we create images on screens. Understanding its core concepts is essential. This includes vertices, buffers, and shaders. Each plays a vital role in what we see.
Think of vertices as points in space. They make up shapes, like dots in a connect-the-dots drawing. When combined, these points create a picture. Each vertex has a position on the screen, which guides how graphics appear. Without vertices, there would be no visible graphics.
Now, let’s talk about shaders. Shaders are small programs that tell the GPU how to process vertices. They control color, lighting, and other effects. Picture a chef adding spices to a dish; shaders enhance the look of graphics. They are crucial for bringing visuals to life. A well-tuned shader can change a dull scene into something vibrant.
Next, we consider buffers. Buffers are like containers for these vertices. They hold the data until the program needs it. Imagine a box filled with pencils. You take them out one at a time to draw. Similarly, buffers store vertex information securely.
- Vertices are the building blocks of shapes.
- Buffers help manage vertex data efficiently.
- Shaders enhance visuals by processing graphic elements.
When a game renders a character, these elements work together. The vertices outline the character’s shape. Buffers keep this information organized. Then, shaders add dynamic touches like shadows or highlights.
Consider a 3D video game. The character moves smoothly. It happens because of these core concepts. Smooth graphics result from the combined effort of vertices, buffers, and shaders. Without one, the display would falter.
To visualize this, think of a painter in action. The artist uses a canvas (the screen), paints (vertices), a palette (buffers), and brushes (shaders). Together, they create a masterpiece.
In applications like video games and simulations, these concepts are Everywhere. They are not just for programmers but also for gamers. Understanding them helps appreciate the magic behind the screen.
Each game developer needs to grasp these ideas. They are foundational to creating interactive experiences. As developers gain skill, mastering these concepts becomes vital.
Creating Your First OpenGL Application: A Step-by-Step Tutorial

Making your first OpenGL app can be exciting. Let’s dive in. OpenGL will help you draw stunning graphics. You will need a few tools to get started.
Step 1: Set Up Your Environment
First, install a text editor. Code needs a properly set up space. Programs like Visual Studio Code work well. You also need to install OpenGL drivers. If you’re on Windows, these drivers often come with graphics card software. Check your manufacturer’s website.
Step 2: Create a New Project
Open your text editor. Create a new folder for your project. We will call this folder “MyFirstOpenGLApp”. Inside it, create a file named main.cpp. This will be where your code lives. Please follow me closely.
Step 3: Write Your Basic Code
Copy and paste the following code into your main.cpp file:
#include <GL/glut.h> void display() { glClear(GL_COLOR_BUFFER_BIT); glBegin(GL_TRIANGLES); glVertex2f(-0.5, -0.5); glVertex2f(0.5, -0.5); glVertex2f(0, 0.5); glEnd(); glFlush(); } int main(int argc, char** argv) { glutInit(&argc, argv); glutCreateWindow("My First OpenGL App"); glutDisplayFunc(display); glutMainLoop(); return 0; }
This code will draw a triangle on the screen. It clears the window and displays the shape. Did you see how it works? Every piece of code plays its part.
Step 4: Compile and Run Your App
Now you must compile your program. Open a terminal window. Navigate into your project folder. Type this command: g++ main.cpp -o MyFirstOpenGLApp -lGL -lGLU -lglut. Hit enter! This should create an executable file.
To run your app, type ./MyFirstOpenGLApp and press enter. If all goes well, a window should appear. This window is your gateway to the world of OpenGL!
Step 5: Experiment and Learn
Change vertex positions. Slip into the shoes of a designer. Modify the colors. Add more shapes. You learn best when you play around. Don’t be afraid to make mistakes!
- Too many errors? Check your syntax.
- Missed libraries? Install them!
- Window doesn’t show? Check your settings.
- Code not compiling? Mind your brackets!
Each issue is a chance to learn. Happy coding will lead you through these challenges. Break a leg!
Common Challenges and Solutions in OpenGL Development
Developers often face challenges when using OpenGL. Different problems arise during rendering. Performance issues are also common. Debugging can be tricky too. Let’s look at some real case studies.
Rendering Issues
Sometimes objects don’t appear on the screen. This can happen due to incorrect blending settings. Check your shader code for mistakes. A developer named Sam found this error while working on his project. He fixed it by adjusting his blend function. Always double-check your blend modes. This simple adjustment made all the difference.
Performance Optimization
Performance is crucial in graphics programming. Slow applications frustrate users. One case involved a game with slow rendering frames. The team struggled until they optimized their vertex buffer. They switched to vertex array objects (VAOs). That improved speed greatly.
- Use efficient data structures.
- Minimize state changes.
- Batch draw calls for efficiency.
Each of these strategies proved helpful. They took patience and practice to implement.
Debugging Challenges
Debugging can feel like finding a needle in a haystack. Errors often seem hidden. A developer named Lisa faced a similar issue. Her textures weren’t loading correctly. After checking multiple layers of code, she discovered a simple typo in her file path. Every little detail matters. This teaches us about the importance of clarity.
Tools like gDEBugger can assist in finding such problems. These tools provide a visual aid. They help catch bugs that are hard to see just by looking at code.
The Importance of Community
The OpenGL community is a useful resource. Many developers share their experiences online. Joining discussions can provide support. They can offer solutions based on their hardships.
Online forums like GitHub have dedicated groups. They discuss everything from simple mistakes to complex challenges. That helps build a strong network.
Perseverance is key in OpenGL development. Keep trying and learning from failures. Each problem teaches us a new lesson. Resourcefulness also helps build better programs.
The Future of OpenGL and Graphics Programming

The world of graphics is rapidly changing. Real-time ray tracing is becoming more popular. It offers lifelike lighting. This technology will reshape our visual experiences. Imagine being immersed in bright worlds. What could this mean for game developers and artists?
They now have more tools at their disposal. Virtual reality (VR) enhances interactions. VR aims to pull users deeper into story.
- How will OpenGL adapt to these changes?
- Will future hardware run more complex shaders?
- Can OpenGL support emerging trends effectively?
Many are questioning where the technology will lead. Graphics hardware continues to advance rapidly. New GPUs bring much more massive calculations. The experience will only grow more lifelike. This may also simplify processes for developers. Learning OpenGL remains significant.
It forms a strong base for understanding graphics. As developers work with OpenGL, they change. They adapt to new features and trends. Future graphics programming may shift towards simplicity. Will the basic principles remain unchanged? At the same time, challenges will persist.
How will developers tackle issues in ray tracing or VR? Collaboration could drive innovative solutions. Many experts believe learning continues to be crucial. The future needs skilled creators. With new tech, they will be prepared. Thus, understanding OpenGL is a wise investment.
Exciting developments are on the horizon. The possibilities are endless, aren’t they? Moreover, recent trends indicate expansion. What new ideas will emerge in graphics programming? Ongoing education will shape tomorrow’s experiences. Those who remain proactive will find success.